5 snippets to differentiate undefined and omitted values in javascript
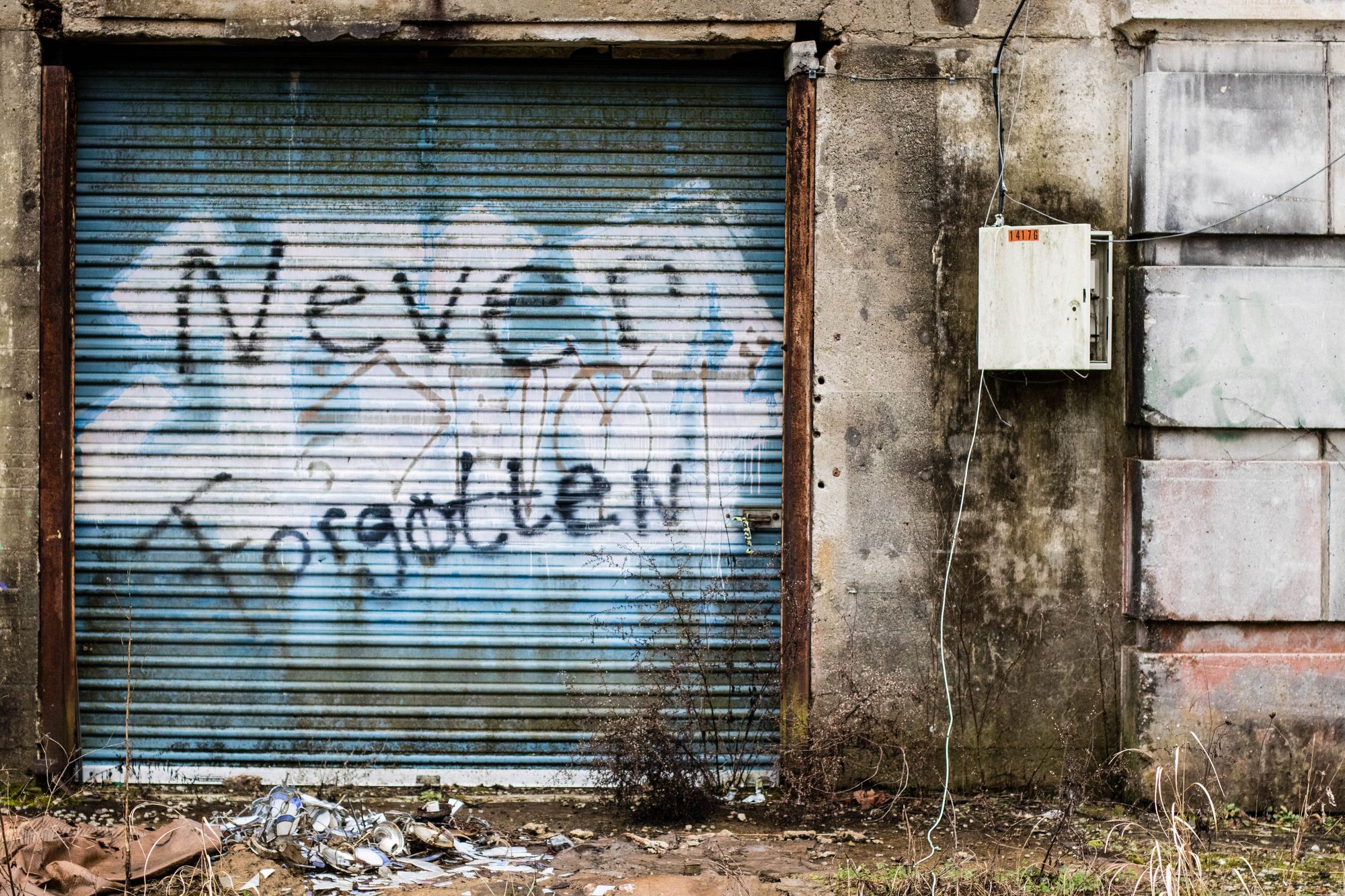
In Javascript, in general, to check if a variable is undefined
or omitted, we usually use typeof x === 'undefined'
. However, sometimes you need to go further and differentiate these two cases, that if a variable is assigned with the undefined
value or is omitted/absent.
This post shows several ways to distinguish between the undefined
and omitted variables in several situations. Suppose that in all these situations, the key is already tested with typeof ... === 'undefined'
. We only focus on distinguish between the omitted value and the undefined
value.
Check a variable
Use the following snippet to check if the variable x
is not defined or defined with the value undefined
.
const check = () => x
let isOmitted = true
try {
check()
isOmitted = false
} catch {}
Check a key in an object
To check if a key key
exist in an object obj
( {}
=> no, {key: undefined}
=> yes)
- Method 1: use
Object.prototype.hasOwnProperty.call(obj, 'key')
(orobj.hasOwnProperty('key')
). This returnstrue
if the value at'key'
is not omitted. - Method 2:
({...{key: 1}, ...obj}).key === undefined
istrue
if and only ifkey
is presented with theundefined
value inobj
. - Method 3:
'key' in obj
returnstrue
iff'key'
key exists inobj
.
Check a value in an array
Use array.length
, Object.prototype.hasOwnProperty
, array.includes
.
// use arr.length
[].length === 0 // true
[undefined].length === 1 // true
// use hasownProperty
const arr = []
arr[1] = undefined
Object.prototype.hasOwnProperty(arr, 0) // false
Object.prototype.hasOwnProperty(arr, 1) // true
// use arr.includes
[].includes() // false
[].includes(undefined) // false
[undefined].includes() // true
[undefined].includes(undefined) // true
Check function parameters
To check if a parameter is not provided or passed with the undefined
value.
Use variadic function parameters and check the position.
const func = (...params) => params.length === 1
// or
// const func = (...params) => Object.prototype.hasOwnProperty(params, 0)
// or
// const func = function(){ return Object.prorotype.hasOwnProperty(parameters, 0) }
func() // returns false
func(undefined) // returns true
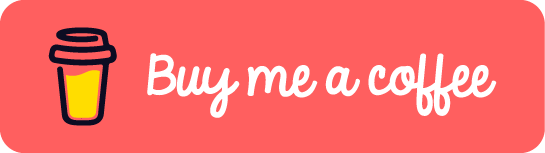