C++ online judge/programming contest template
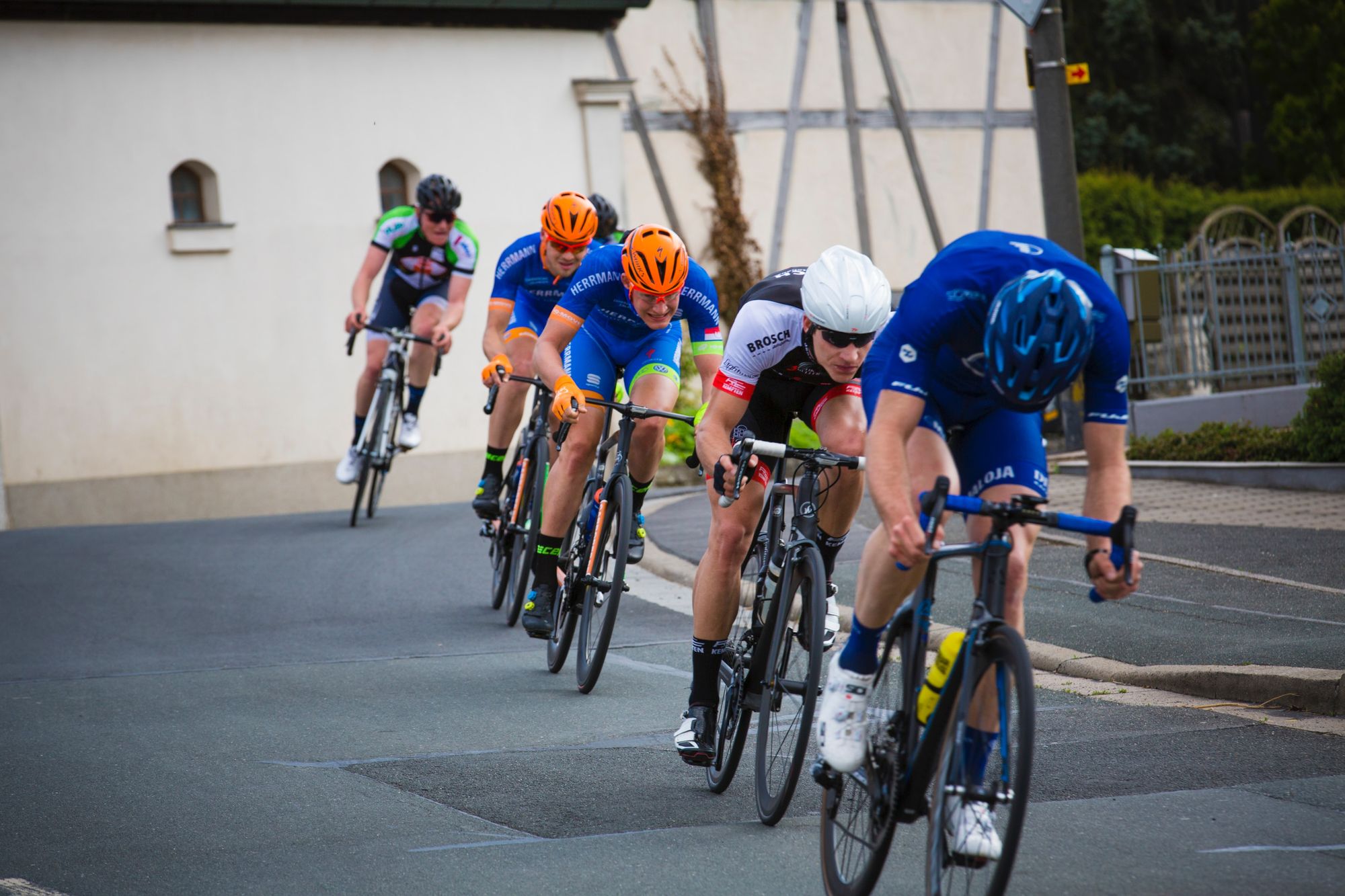
Timing is critical in programming contests. A well-prepared template will help you save the implementation cost once the solution is ready.
This is my template
#include <bits/stdc++.h>
using namespace std;
#define V vector
#define For(i,a,b) for(auto (i) = (a); (i) < (b); (i)++)
#define rep(i,n) For(i,0,(n))
#define mt make_tuple
#define eb emplace_back
#define all(a) (a).begin(), (a).end()
#define ll long long
int main(){
ios::sync_with_stdio(false); cin.tie(nullptr); cout.tie(nullptr);
cout << "hello";
return 0;
}
#include <bits/stdc++.h>
includes everything you need ( algorithm
, iostream
, cstdlib
, vector
, ...)
ios::sync_with_stdio(false); cin.tie(NULL); cout.tie(NULL);
speeds up cin
, cout
commands. These instructions disable the synchronization between stdin/stdout buffer (used in scanf
, printf
) and iostream's buffers. They make cin
, cout
achieve similar performance as scanf
, printf
. Note that you should never mix cin
and scanf
(or cout
with printf
) once you applied these instructions.
In addition
- To scan a space/tab-separated string, use
string str; cin >> str;
. - To scan a whole line, use
string str; getline(cin, str);
(newline character\n
is not included). - To print float/double with pre-defined precision, use
double d = 3.1415926534;
cout << hexfloat << d << ' ' //binary presentation: 0x1.921fb543da7a8p+1
<< scientific << setprecision(2) << d << ' ' //2 decimal digits: 3.14e+00
<< fixed << setprecision(3) << d << ' ' //3 decimal digits: 3.142
<< scientific << setprecision(11) << d << ' ' //3.14159265340e+00
<< fixed << setprecision(12) << d << ' ' //3.141592653400
<< defaultfloat << d << '\n'; // 3.1415926534
// '\n' has a bit higher performance compared to endl
- To print bit presentation of int or long long int
cout << bitset<32>(int_val);
. Note thatdouble
value is cast toint
. - To redirect input/output from/to files. Use shell redirection, for example:
./main <input.txt >output.txt
, or, in code, use:
freopen("input.txt", "r", stdin);
freopen("output.txt", "w", stdout);
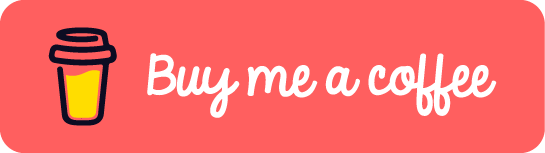