How to break/continue the outer loop from an inner loop in javascript
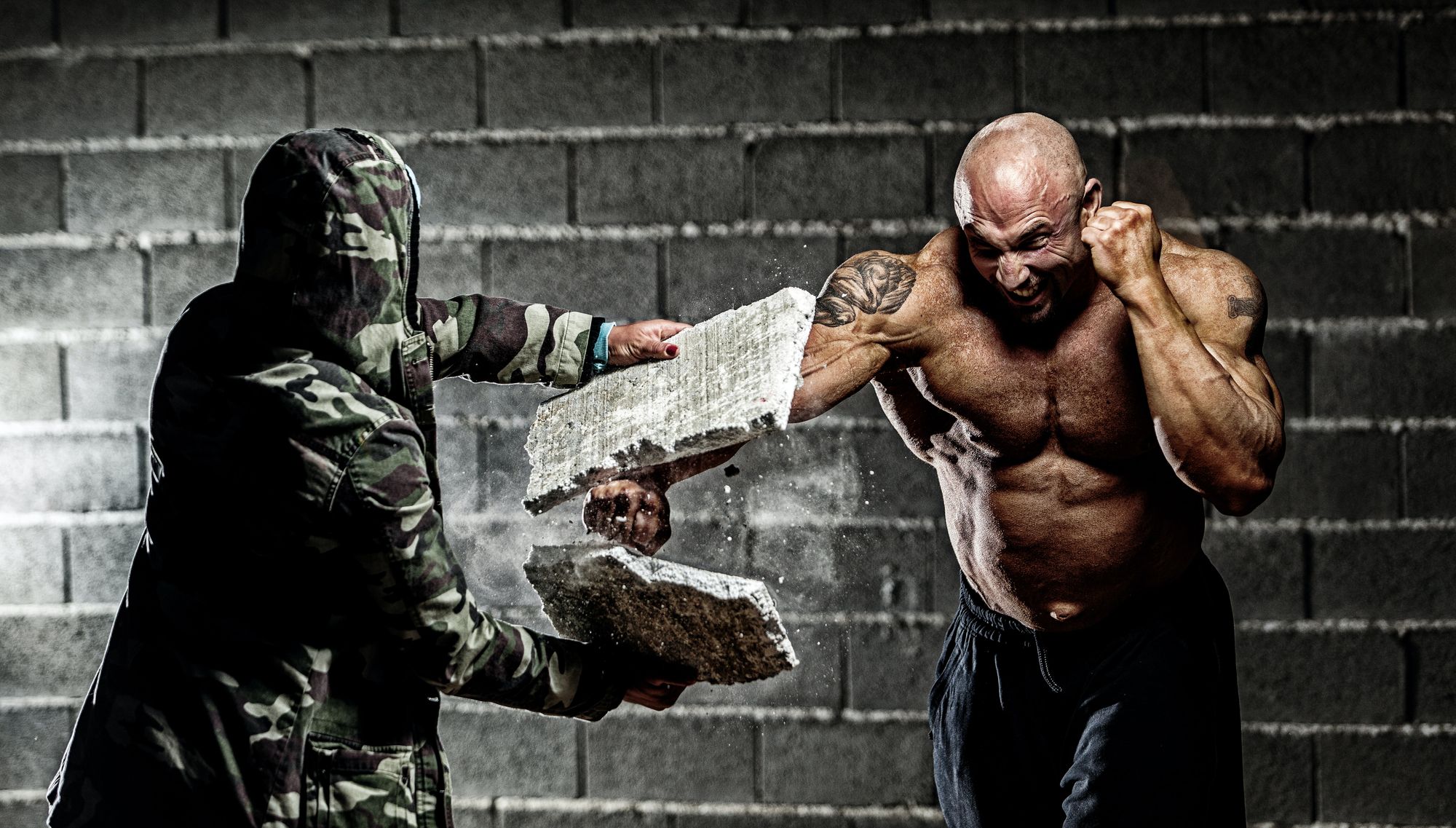
When it happens that you want to find the first pair of elements from two arrays. You would want to break the for-loop after the predicate is satisfied.
The common approach is to introduce a flag and check after each iteration in the outer loop.
// NOTE: we have a better version of this code
let found = false
for (const a of arrayA) {
for (const b of arrayB)
if (predicate(a, b)) {
found = true
break
}
if (found) break
}
With the label statement syntax, we can have a much better solution.
let found = false
aLoop:
for (const a of arrayA)
for (const b of arrayB)
if (predicate(a, b)) {
found = true
break aLoop
}
This is very useful especially when there are more than 2 loops nested because we can easily specify which loop to break from any nested level.
Also, a label can be used with continue
, such as continue aLoop
Block without a for-loop can have a label, but this label can be used with break
only (not continue
. For instance:
myBlock: {
console.log('a')
if (aCondition) break myBlock
console.log('b')
}
This is useful when you have many if/else conditions, and you want to avoid using nested if/else or functions to make the code cleaner.
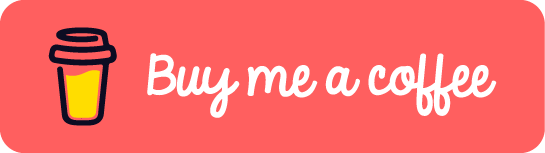