Write react code without JSX
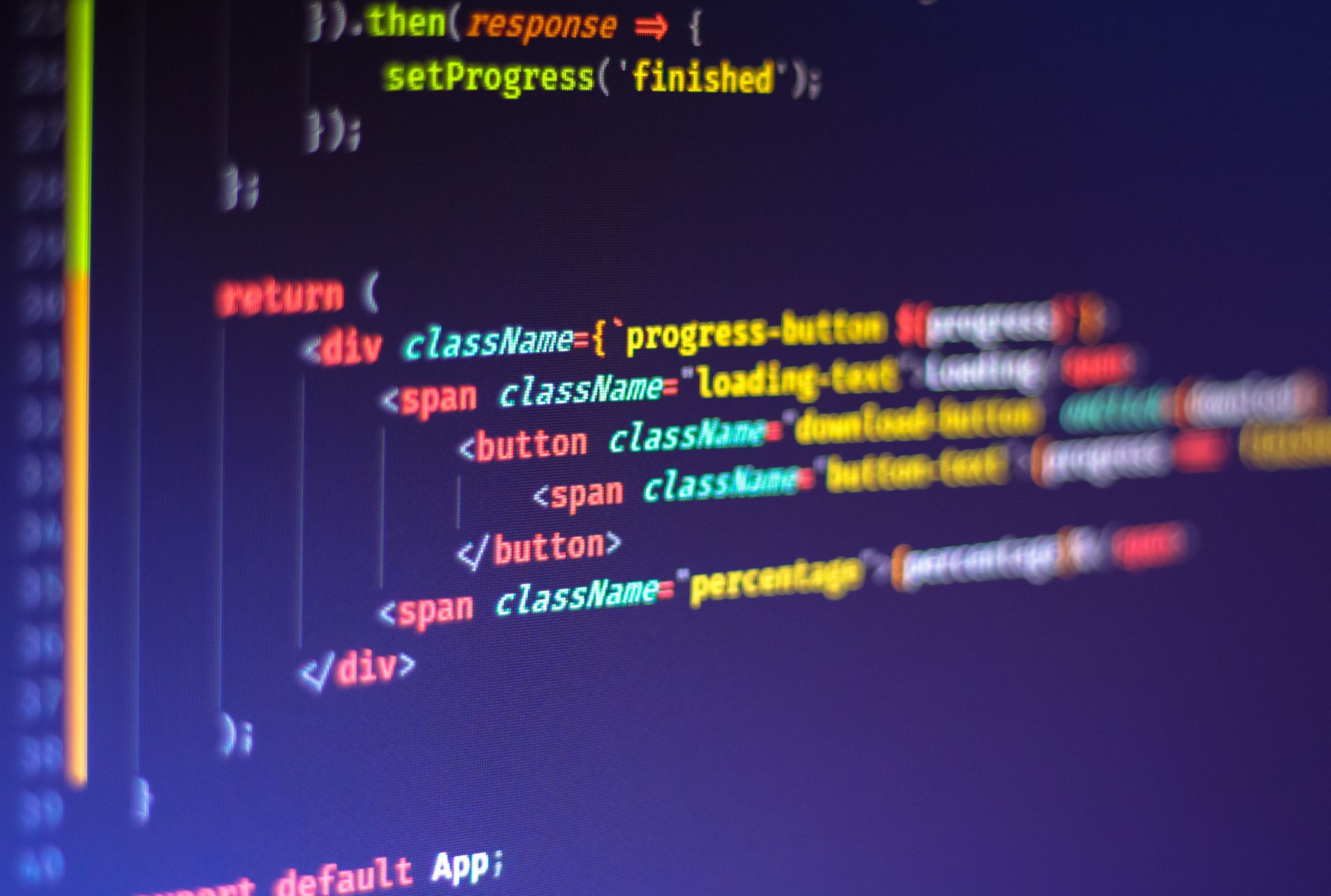
All following snippets are equivalent
const React = require('react')
//or import React from 'react'
const element = React.createElement('div', {id: 'my-div'}, 'hello world')
const element = <div id="my-div">hello world</div>
const element = {
$$typeof: Symbol.for('react.element'),
type: 'div',
props: {
id: 'my-div',
children: 'hello world'
}
}
'div'
can be replaced with react component definition (component class or functional component).
The last method is not recommended, as react element definition can be changed in the future.
In general, <Comp {...props}>{child}</Comp>
is transpiled to
createElement(
Comp,
props,
child
)
If Comp
is HTML primary tag, it is replaced by the string value whose value is the tag name.
Source:
React Components, Elements, and Instances – React Blog
The difference between components, their instances, and elements confuses many React beginners. Why are there three different terms to refer to something that is painted on screen? Managing the Instances If you’re new to React, you probably only worked with component classes and instances before.…

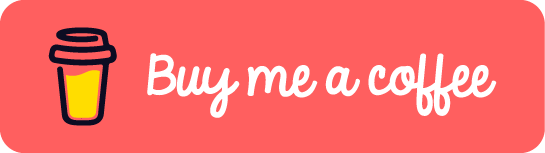